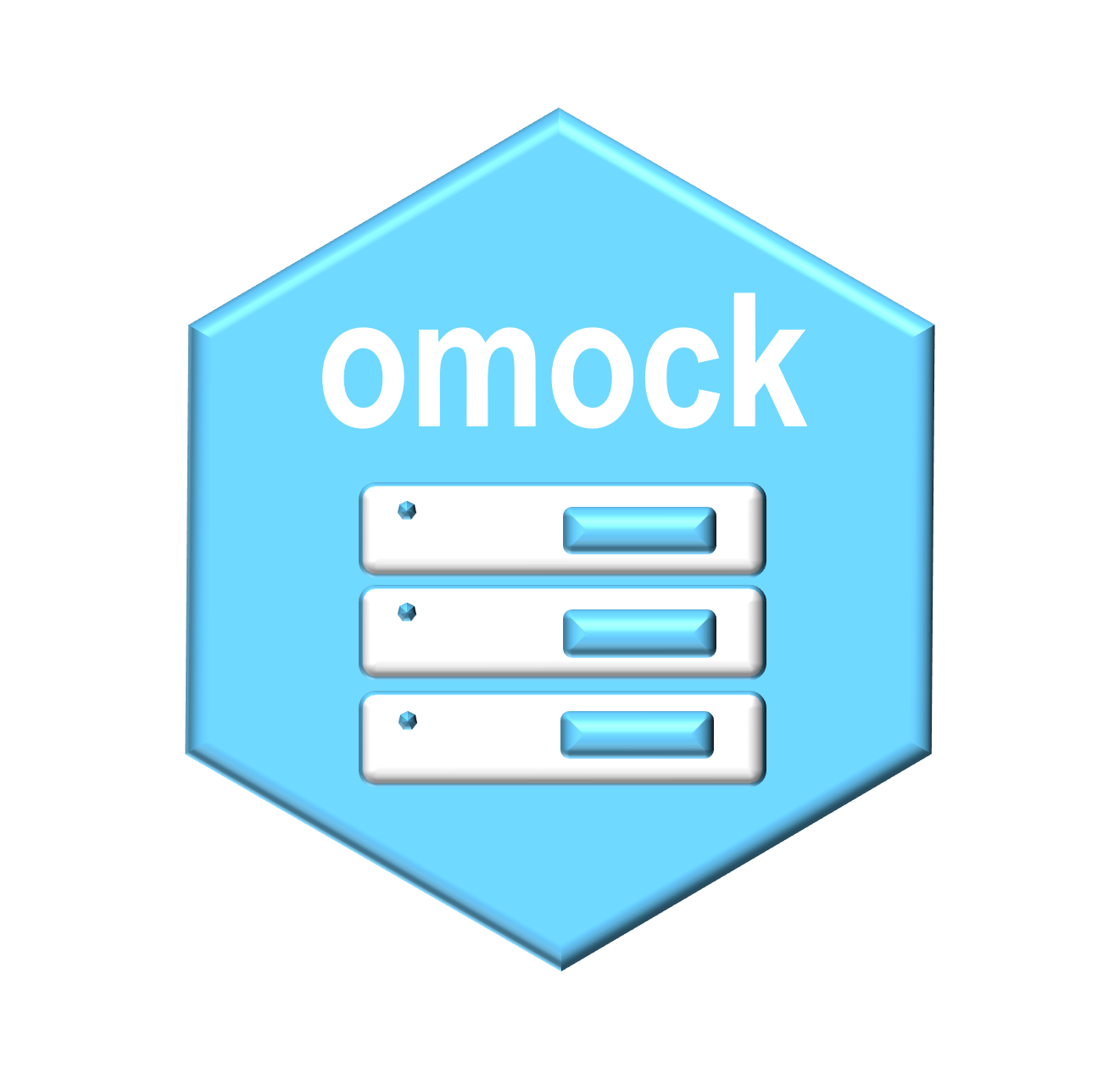
Generates a mock observation period table and integrates it into an existing CDM object.
Source:R/mockObservationPeriod.R
mockObservationPeriod.Rd
This function simulates observation periods for individuals based on their date of birth recorded in the 'person' table of the CDM object. It assigns random start and end dates for each observation period within a realistic timeframe up to a specified or default maximum date.
Arguments
- cdm
A `cdm_reference` object that must include a 'person' table with valid dates of birth. This object serves as the base CDM structure where the observation period data will be added. The function checks to ensure that the 'person' table is populated and uses the date of birth to generate observation periods.
- seed
An optional integer used to set the seed for random number generation, ensuring reproducibility of the generated data. If provided, this seed allows the function to produce consistent results each time it is run with the same inputs. If 'NULL', the seed is not set, which can lead to different outputs on each run.
Value
Returns the modified `cdm` object with the new 'observation_period' table added. This table includes the simulated observation periods for each person, ensuring that each record spans a realistic timeframe based on the person's date of birth.
Examples
library(omock)
# Create a mock CDM reference and add observation periods
cdm <- mockCdmReference() |>
mockPerson(nPerson = 100) |>
mockObservationPeriod()
# View the generated observation period data
print(cdm$observation_period)
#> # A tibble: 100 × 5
#> observation_period_id person_id observation_period_s…¹ observation_period_e…²
#> * <int> <int> <date> <date>
#> 1 1 1 1994-01-05 2013-03-06
#> 2 2 2 1991-04-14 2013-12-10
#> 3 3 3 2003-11-12 2011-04-26
#> 4 4 4 1975-03-01 2010-10-05
#> 5 5 5 2014-06-28 2018-10-05
#> 6 6 6 2016-02-15 2017-09-14
#> 7 7 7 1981-01-12 2019-05-03
#> 8 8 8 2004-04-14 2017-03-19
#> 9 9 9 2011-11-11 2015-05-15
#> 10 10 10 2003-02-21 2009-07-25
#> # ℹ 90 more rows
#> # ℹ abbreviated names: ¹observation_period_start_date,
#> # ²observation_period_end_date
#> # ℹ 1 more variable: period_type_concept_id <int>