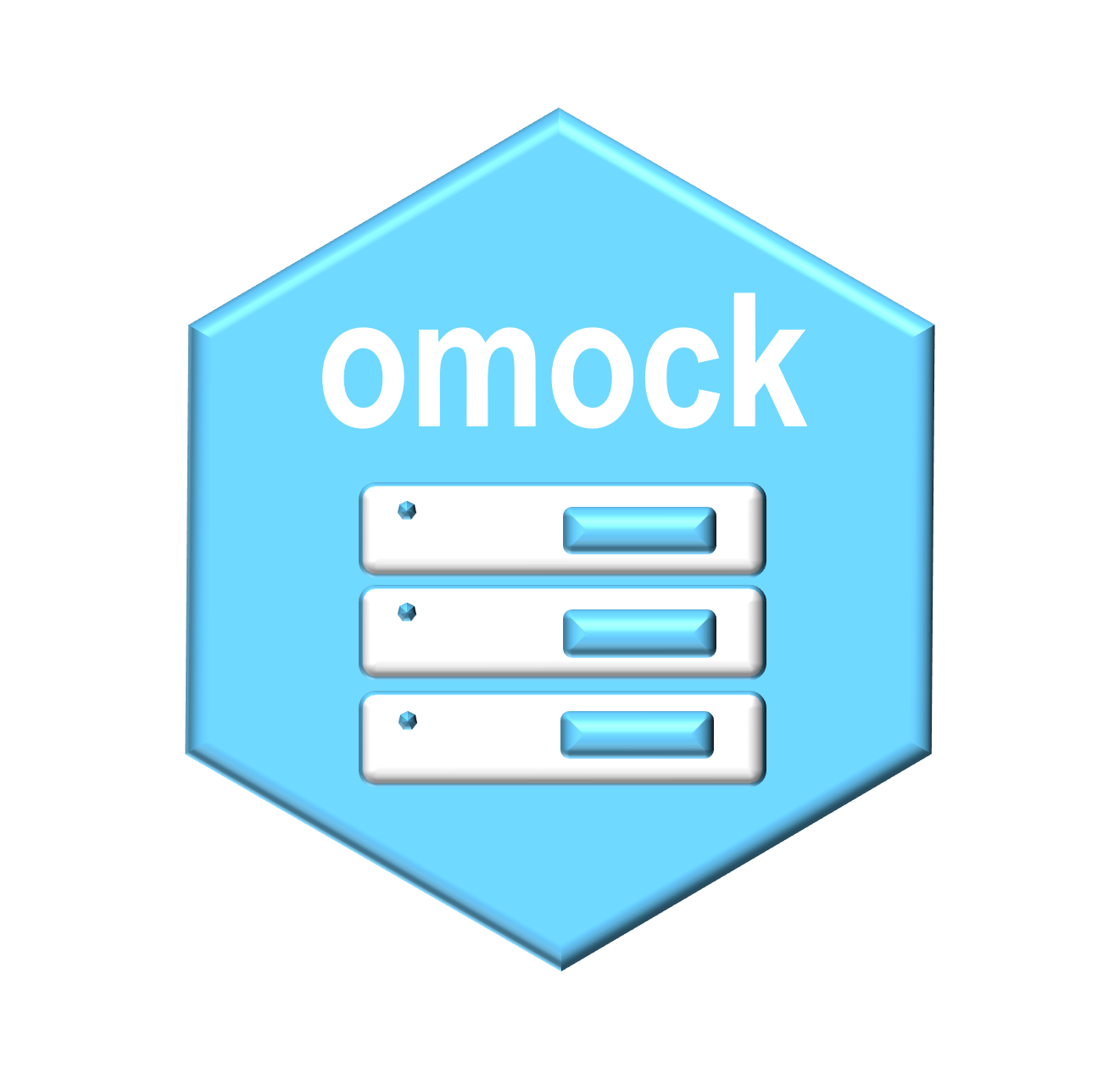
Generates a mock observation table and integrates it into an existing CDM object.
Source:R/mockObservation.R
mockObservation.Rd
This function simulates observation records for individuals within a specified cohort. It creates a realistic dataset by generating observation records based on the specified number of records per person. Each observation record is correctly associated with an individual within valid observation periods, ensuring the integrity of the data.
Arguments
- cdm
A `cdm_reference` object that must already include 'person', 'observation_period', and 'concept' tables. This object serves as the base CDM structure where the observation data will be added. The 'person' and 'observation_period' tables must be populated as they are necessary for generating accurate observation records.
- recordPerson
An integer specifying the expected number of observation records to generate per person. This parameter allows for the simulation of varying frequencies of healthcare observations among individuals in the cohort, reflecting real-world variability in patient monitoring and health assessments.
- seed
An optional integer used to set the seed for random number generation, ensuring reproducibility of the generated data. If provided, this seed enables the function to produce consistent results each time it is run with the same inputs. If 'NULL', the seed is not set, which can lead to different outputs on each run.
Value
Returns the modified `cdm` object with the new 'observation' table added. This table includes the simulated observation data for each person, ensuring that each record is correctly linked to individuals in the 'person' table and falls within valid observation periods.
Examples
library(omock)
# Create a mock CDM reference and add observation records
cdm <- mockCdmReference() |>
mockPerson() |>
mockObservationPeriod() |>
mockObservation(recordPerson = 3)
# View the generated observation data
print(cdm$observation)
#> # A tibble: 30 × 18
#> observation_concept_id person_id observation_date observation_id
#> * <int> <int> <date> <int>
#> 1 9 7 2000-01-09 1
#> 2 9 3 1991-11-19 2
#> 3 9 7 2001-01-01 3
#> 4 9 6 2002-07-15 4
#> 5 9 9 1997-06-10 5
#> 6 9 1 2013-01-06 6
#> 7 9 9 1993-11-22 7
#> 8 9 3 1983-01-19 8
#> 9 9 10 2016-07-17 9
#> 10 9 9 1994-03-07 10
#> # ℹ 20 more rows
#> # ℹ 14 more variables: observation_type_concept_id <int>,
#> # observation_datetime <dttm>, value_as_number <dbl>, value_as_string <chr>,
#> # value_as_concept_id <int>, qualifier_concept_id <int>,
#> # unit_concept_id <int>, provider_id <int>, visit_occurrence_id <int>,
#> # visit_detail_id <int>, observation_source_value <chr>,
#> # observation_source_concept_id <int>, unit_source_value <chr>, …