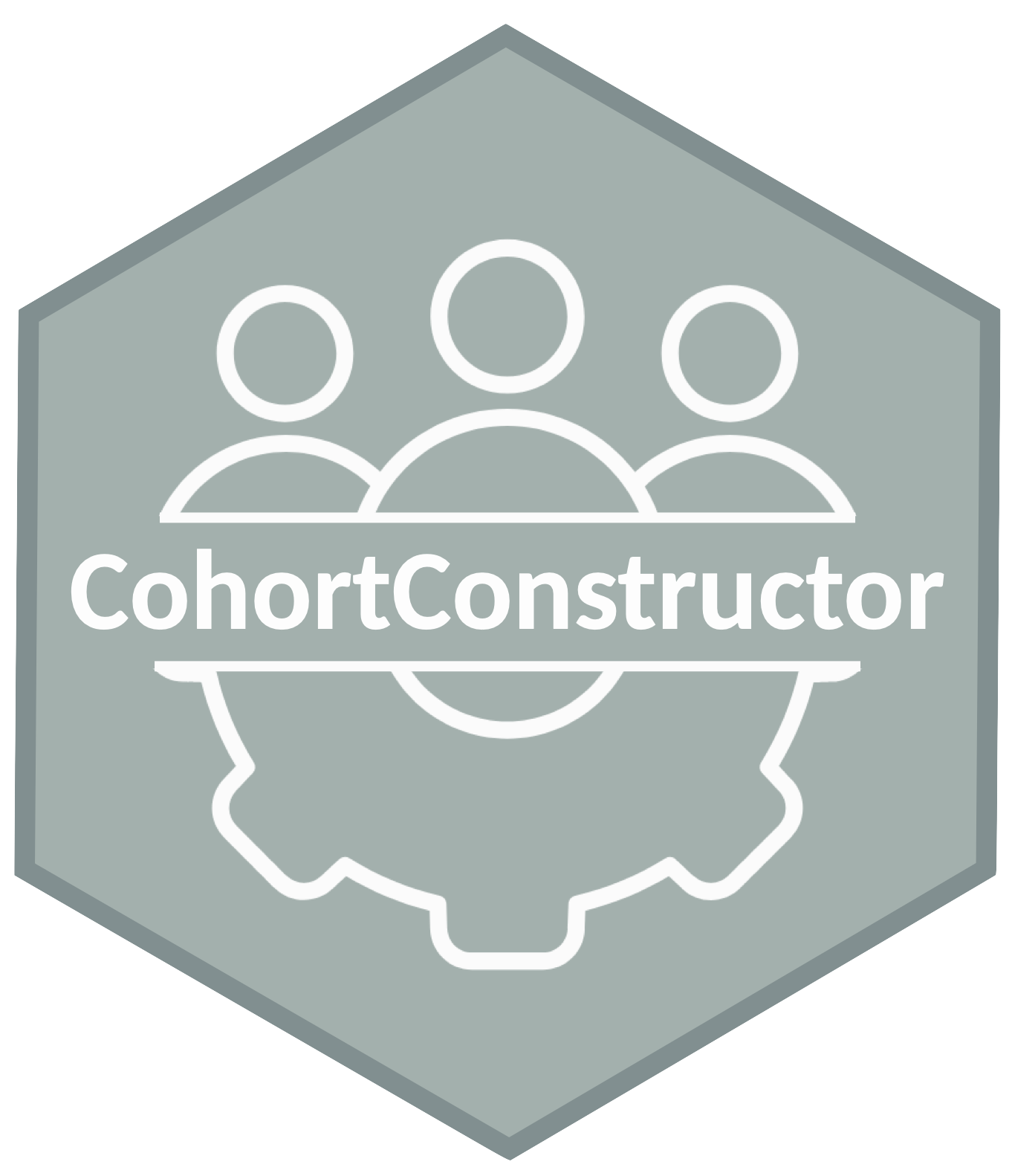
Set cohort end date to the first of a set of column dates
Source:R/exitAtColumnDate.R
exitAtFirstDate.Rd
exitAtFirstDate()
resets cohort end date based on a set of specified
column dates. The first date that occurs is chosen.
Usage
exitAtFirstDate(
cohort,
dateColumns,
cohortId = NULL,
returnReason = TRUE,
name = tableName(cohort)
)
Arguments
- cohort
A cohort table in a cdm reference.
- dateColumns
Character vector indicating date columns in the cohort table to consider.
- cohortId
Vector identifying which cohorts to modify (cohort_definition_id or cohort_name). If NULL, all cohorts will be used; otherwise, only the specified cohorts will be modified, and the rest will remain unchanged.
- returnReason
If TRUE it will return a column indicating which of the
dateColumns
was used.- name
Name of the new cohort table created in the cdm object.
Examples
# \donttest{
library(CohortConstructor)
cdm <- mockCohortConstructor(tables = list(
"cohort" = dplyr::tibble(
cohort_definition_id = 1,
subject_id = c(1, 2, 3, 4),
cohort_start_date = as.Date(c("2000-06-03", "2000-01-01", "2015-01-15", "2000-12-09")),
cohort_end_date = as.Date(c("2001-09-01", "2001-01-12", "2015-02-15", "2002-12-09")),
date_1 = as.Date(c("2001-08-01", "2001-01-01", "2015-01-15", "2002-12-09")),
date_2 = as.Date(c("2001-08-01", NA, "2015-04-15", "2002-12-09"))
)
))
cdm$cohort |> exitAtFirstDate(dateColumns = c("date_1", "date_2"))
#> # Source: table<main.cohort> [?? x 5]
#> # Database: DuckDB v1.1.3 [unknown@Linux 6.8.0-1020-azure:R 4.4.2/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date exit_reason
#> <int> <int> <date> <date> <chr>
#> 1 1 2 2000-01-01 2001-01-01 date_1
#> 2 1 4 2000-12-09 2002-12-09 date_1; dat…
#> 3 1 1 2000-06-03 2001-08-01 date_1; dat…
#> 4 1 3 2015-01-15 2015-01-15 date_1
# }