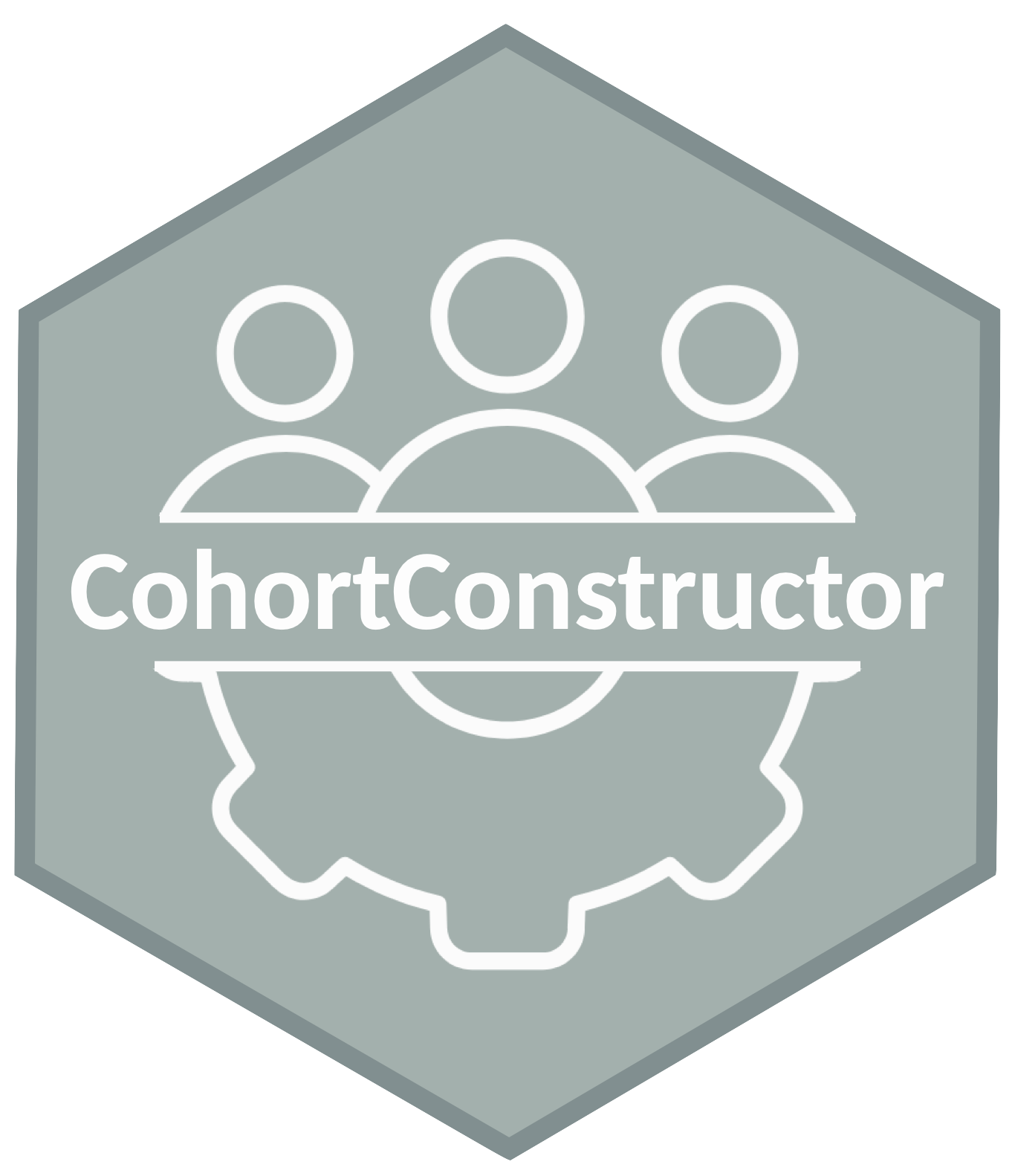
Create a new cohort table from stratifying an existing one
Source:R/stratifyCohorts.R
stratifyCohorts.Rd
stratifyCohorts()
creates new cohorts, splitting an existing cohort based
on specified columns on which to stratify on.
Usage
stratifyCohorts(
cohort,
strata,
cohortId = NULL,
removeStrata = TRUE,
name = tableName(cohort)
)
Arguments
- cohort
A cohort table in a cdm reference.
- strata
A strata list that point to columns in cohort table.
- cohortId
Vector identifying which cohorts to include (cohort_definition_id or cohort_name). Cohorts not included will be removed from the cohort set.
- removeStrata
Whether to remove strata columns from final cohort table.
- name
Name of the new cohort table created in the cdm object.
Examples
# \donttest{
library(CohortConstructor)
library(PatientProfiles)
cdm <- mockCohortConstructor()
cdm$my_cohort <- cdm$cohort1 |>
addAge(ageGroup = list("child" = c(0, 17), "adult" = c(18, Inf))) |>
addSex(name = "my_cohort") |>
stratifyCohorts(
strata = list("sex", c("sex", "age_group")), name = "my_cohort"
)
cdm$my_cohort
#> # Source: table<my_cohort> [?? x 5]
#> # Database: DuckDB v1.2.0 [unknown@Linux 6.8.0-1021-azure:R 4.4.2/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date age
#> <int> <int> <date> <date> <int>
#> 1 1 2 1964-09-18 1965-08-30 9
#> 2 1 3 1976-11-28 1977-03-11 17
#> 3 1 4 1998-06-22 2001-02-12 16
#> 4 1 5 2007-10-19 2008-11-10 44
#> 5 2 6 2003-11-15 2004-04-10 35
#> 6 1 9 2012-01-18 2012-03-08 27
#> 7 1 3 1978-04-04 1987-02-26 19
#> 8 1 5 2008-11-11 2011-09-12 45
#> 9 2 6 2003-10-31 2003-11-14 35
#> 10 1 3 1977-03-12 1978-04-03 17
#> # ℹ more rows
settings(cdm$my_cohort)
#> # A tibble: 6 × 8
#> cohort_definition_id cohort_name target_cohort_id target_cohort_name
#> <int> <chr> <int> <chr>
#> 1 1 cohort_1_female 1 cohort_1
#> 2 2 cohort_1_male 1 cohort_1
#> 3 3 cohort_1_female_adult 1 cohort_1
#> 4 4 cohort_1_female_child 1 cohort_1
#> 5 5 cohort_1_male_adult 1 cohort_1
#> 6 6 cohort_1_male_child 1 cohort_1
#> # ℹ 4 more variables: target_cohort_table_name <chr>, strata_columns <chr>,
#> # sex <chr>, age_group <chr>
attrition(cdm$my_cohort)
#> # A tibble: 16 × 7
#> cohort_definition_id number_records number_subjects reason_id reason
#> <int> <int> <int> <int> <chr>
#> 1 1 10 6 1 Initial qualif…
#> 2 1 8 6 2 filter strata:…
#> 3 2 10 6 1 Initial qualif…
#> 4 2 2 1 2 filter strata:…
#> 5 3 10 6 1 Initial qualif…
#> 6 3 8 6 2 filter strata:…
#> 7 3 4 3 3 filter strata:…
#> 8 4 10 6 1 Initial qualif…
#> 9 4 8 6 2 filter strata:…
#> 10 4 4 3 3 filter strata:…
#> 11 5 10 6 1 Initial qualif…
#> 12 5 2 1 2 filter strata:…
#> 13 5 2 1 3 filter strata:…
#> 14 6 10 6 1 Initial qualif…
#> 15 6 2 1 2 filter strata:…
#> 16 6 0 0 3 filter strata:…
#> # ℹ 2 more variables: excluded_records <int>, excluded_subjects <int>
# }